During my weekends/time off I tend to watch quite a lot of sport, with the English Premier League (EPL) being one of my favourite things to support. As such, I created and run an EPL facebook page dedicated to covering fixtures, results, and other news.
Now this all good fun and I enjoy doing it, but week after week, month after month of posting all the fixtures the day before and posting the results the day after gets a little tedious, especially if I’m short on time. So being the geek I am, I decided that I could easily automate these, and so I did, using the PHP facebook SDK.
It was actually pretty straight forward, the hardest bit was trying to find websites which would give me the information in a straight forward and easy way. In the end, I decided to go with the offical Premier League website, as not only is it the correct information it is also laid out in a way which makes it easy to retrieve.
So putting my previous posts into good use I used curl to scrape the website and Xpath to dissect it into the important parts (namely the home and away teams, the location, and the final score).
Once I have this important information I can then go away and use the PHP facebook SDK to post a status to my EPL News feed which I will show you how to do now.
Firstly, you will need to create a facebook application which will be integrated in a website. After you’ve created the app you will want to fill out the website URL and hit save. This URL is going to be where the PHP app you are going to create will be stored (ie http://devhour.net/fbapp).
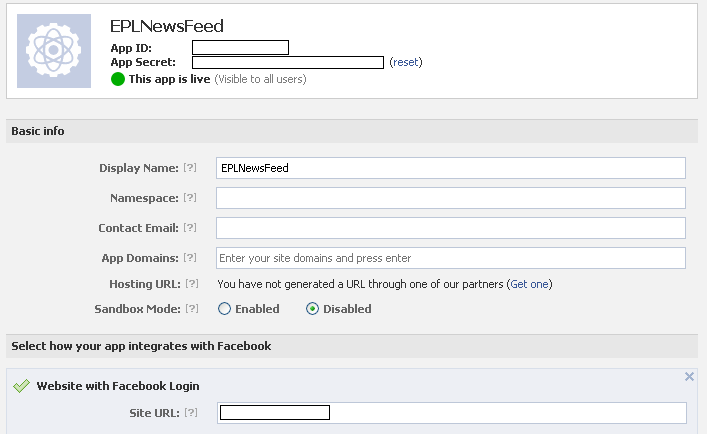
Next up you’ll need to run the following URL to authorize your app to manage your pages and other such permissions. Remember to replace [APP_ID] and [SITE_URL] with both your facebook APP ID and the website you entered above. You will be prompted to grant access to your app (click Allow).
https://graph.facebook.com/oauth/authorize?client_id=[APP_ID]&scope=offline_access,publish_stream,manage_pages&redirect_uri=[SITE_URL]
It will then redirect you back to the URL of the site you specified in the app. If you look closely though you’ll notice that on the end of the url is ?code=aBuNcHoFgIbBeRiSh. Copy everything after the ?code= and keep it in a safe place for now. You’re going to need it in a second.
The next step is to get the long lasting access token which will allow you to keep posting without having to authenticate yourself every time (this is vital to making this whole script automatic). So again go to the following URL and replace [APP_ID], [APP_SECRET], [SITE_URL], and [CODE] (the one you just previously copied).
https://graph.facebook.com/oauth/access_token?client_id=[APP_ID]&redirect_uri=[SITE_URL]&client_secret=[APP_SECRET]&code=[CODE]
If all went well you’ll be redirected back to your website and on the screen you should see:
access_token=…….
Store this somewhere because it’s very important 🙂
Now the fun can start. Firstly, you can test the access token by going to the Facebook Graph API explorer, pasting the token into the Access token field and trying the following:
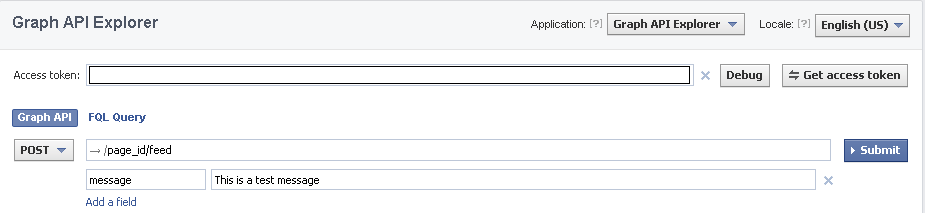
This will post a test message to your page which will indicate that the access token you have is good to go. Now on to the code…
Before we write any code on our end, you’re going to have to download the PHP facebook SDK from the github repo. Download and store these files somewhere handy and feel free to jump into the examples are have a bit of a play.
On our end however, the first thing we are going to is create a php file and reference the facebook sdk and enter some variable details (fill out as necessary).
<?php
require "src/facebook.php";
$PAGE_ID = ""; // The page you want to post to (you must be a manager)
$FACEBOOK_APP_ID = ""; // Your facebook app ID
$FACEBOOK_SECRET = ""; // Your facebook secret
$ACCESS_TOKEN = ""; // The access token you receieved above
$PAGE_TOKEN = ""; // Leave this blank. It will be set later
PHPNow we go ahead and create a new facebook instance using our newly created app details.
$facebook = new Facebook(array('appId' => $FACEBOOK_APP_ID, 'secret' => $FACEBOOK_SECRET, 'cookie' => true,));
$post = array('access_token' => $ACCESS_TOKEN);
PHPThe next little piece of code will loop through the pages that you manage and find the PAGE_TOKEN which matches the PAGE_ID you specified. This will allow you to post to that pages wall as the page rather than you.
try {
$res = $facebook->api('/me/accounts','GET',$post);
if (isset($res['data'])) {
foreach ($res['data'] as $account) {
if ($PAGE_ID == $account['id']) {
$PAGE_TOKEN = $account['access_token'];
break;
}
}
}
}
catch (Exception $e){
echo $e->getMessage();
}
PHPTaking all the information we have so far received we can now go ahead and post to the pages wall.
$message = "This is a test message";
$post = array('access_token' => $PAGE_TOKEN, 'message' => $message);
try{
$res = $facebook->api("/$PAGE_ID/feed","POST",$post);
}
catch (Exception $e){
echo $e->getMessage();
}
?>
PHPAnd that’s it. It’s actually really straight forward and easy to do. Feel free to play around with it and I’d be interested in seeing what you can come up with.
As always, follow me on twitter: @JAGracie